HTML Canvas Circles
The arc() Method
The arc()
method is used to define a circle.
The arc()
method has the following parameters:
Parameter | Description |
---|---|
x | Required. The x-coordinate of the arc's center |
y | Required. The y-coordinate of the arc's center |
radius | Required. The radius of the arc |
startAngle | Required. The angle where the arc starts in radians |
endAngle | Required. The angle where the arc ends in radians |
counterclockwise | Optional. A boolean value. If set to true, it draws the arc counter-clockwise between the start and end angles. The default is false (clockwise) |
Draw a Full Circle
We can create a full circle with the arc()
method by defining the startAngle as 0 and the endAngle
as 2 * PI:
To draw a circle on the canvas, use the following methods:
-
beginPath()
- Begin a path -
arc()
- Define a circle -
stroke()
- Draw it
Example
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.arc(95, 50, 40, 0, 2 * Math.PI);
ctx.stroke();
</script>
Try it Yourself »
Draw a Full Circle with Colors
Add a fill-color and a stroke-color to the circle:
Example
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.arc(95, 50, 40, 0, 2 * Math.PI);
ctx.fillStyle
= "red";
ctx.fill();
ctx.lineWidth = 4;
ctx.strokeStyle = "blue";
ctx.stroke();
</script>
Try it Yourself »
Draw a Half Circle
Here we change the endAngle to PI (not 2 * PI):
Example
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.arc(95, 50, 40, 0, Math.PI);
ctx.fillStyle
= "red";
ctx.fill();
ctx.stroke();
</script>
Try it Yourself »
More About the Angles of an Arc
The following image shows some of the angles in an arc:
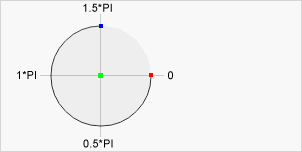
Example
Here we want to draw an arc from start-angle 0 to end-angle 0.5 * PI:
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.arc(95, 50, 40, 0, 0.5 * Math.PI);
ctx.stroke();
</script>
Try it Yourself »
Example
Here we do the same, but with the counterclockwise parameter set to true (it then draws the arc counter-clockwise between the start and end angle):
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.arc(95, 50, 40, 0, 0.5 * Math.PI, true);
ctx.stroke();
</script>
Try it Yourself »